Functions and Scope
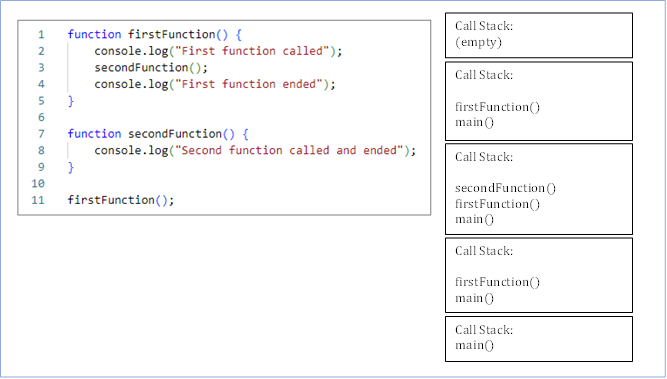
Functions are fundamental building blocks in programming languages, allowing developers to write reusable and modular code. This article will discuss the concept of functions, their importance, and how they interact with scope in JavaScript. Additionally, we will explore execution stacks, queue stacks, and other related topics to help you better understand the inner workings of functions.
Functions in Programming Languages
A function is a self-contained block of code designed to perform a specific task. Functions can be called multiple times with different inputs, making code more modular and easier to maintain. In JavaScript, functions can be defined using the `function` keyword, followed by the function name, a list of parameters within parentheses, and a set of curly braces containing the function body.
Functions can also be defined using arrow functions, which have a shorter syntax and different scoping behavior for the `this` keyword.
Scope in Programming Languages
Scope refers to the visibility and accessibility of variables within a program. In JavaScript, there are two types of scope: global scope and local scope. Variables declared outside of any function or block have a global scope and can be accessed from any part of the code. In contrast, variables declared within a function or block have a local scope and can only be accessed from within that function or block.
Execution Context and Call Stack
When a JavaScript program runs, it creates an execution context that keeps track of the current state of the code, including variables, functions, and the call stack. The call stack is a data structure that stores information about the currently executing functions in a last-in, first-out (LIFO) order. Each time a function is called, a new stack frame is added to the call stack, containing information about the function's local variables, parameters, and return address. When a function finishes executing, its stack frame is removed from the call stack, and the program continues executing from the return address.
Event Loop and Queue Stack
The event loop is a mechanism that processes queued tasks and events in JavaScript. It constantly checks if there are any tasks in the queue stack, which is a data structure that stores tasks in a first-in, first-out (FIFO) order. When a task is ready to be executed, the event loop removes it from the queue stack and adds it to the call stack. This process allows JavaScript to handle asynchronous tasks, such as setTimeout and promises, without blocking the main thread of execution.
In the example above, the output will be:
The second function, which includes a setTimeout, does not block the execution of the third function. The event loop adds the setTimeout callback to the queue stack, and it is executed after the specified delay when the call stack is empty.
Example
- Consider the following JavaScript code:Loading...
- What will be printed to the console?
- Which variables are accessible from the inner function?
- Which variables are accessible from the outer function?
Solution
a) The console will print '10 20 30'.
b) The inner function has access to variables x, y, and z due to its scope.
c) The outer function has access to variables x and y.
Function Parameters and Arguments
Functions can accept input values called parameters, which are declared in the function definition. When a function is called, the values passed into it are called arguments. Arguments are matched to the corresponding parameters in the order they are defined.
Higher-Order Functions and Callbacks
In JavaScript, functions are first-class objects, meaning they can be treated like any other object, such as strings or numbers. This allows for higher-order functions, which are functions that accept other functions as arguments or return them as output. Callback functions are functions passed as arguments to other functions and are often used in asynchronous operations or event handling.
Recursion and the Base Case
Recursion is a programming technique in which a function calls itself to solve a problem. A recursive function typically consists of a base case, which is a condition that ends the recursion, and a recursive case, which is the part of the function that calls itself with a modified argument. Recursive functions can be an elegant solution for certain problems, such as traversing tree-like data structures or solving mathematical problems like the Fibonacci sequence.
Conclusion
Understanding functions and scope in programming languages is essential for writing clean, maintainable code. By learning about execution context, call stacks, and event loops, you can better comprehend the inner workings of functions and the JavaScript runtime. Mastering these concepts will help you become a more effective programmer and problem solver.